FrameIT API Documentation
FrameIT transforms any image into a 3D object to display it in Augmented Reality on mobile devices. FrameIT incorporates a variety of frame styles and colors, giving users the opportunity to ensure that not only the size and style of an artwork, but also its framing, harmonize with their interior.
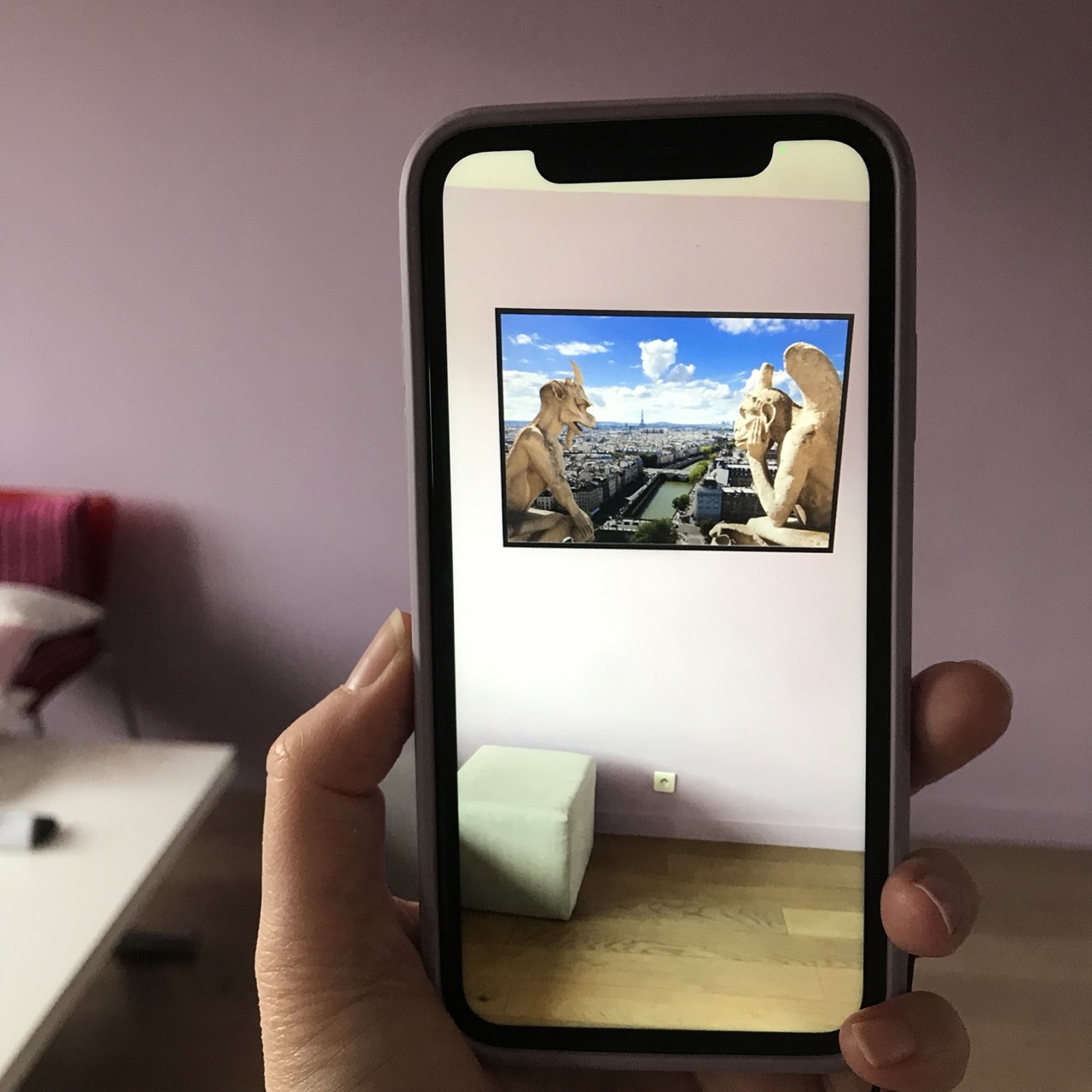
API DEMO
Explore two practical implementations on our Demo page:
- Online Store
- Art Gallery
You can access the demonstrations here.
PARAMETERS OF THE FrameIT API
Base URL of the API: https://www.frameit.ar/api/frameit
Endpoint : /
Description : Adds a frame to an image.
HTTP Method : POST
Request Parameters:
PARAMETER | DESCRIPTION | VALUES (can be extended upon request) |
File | Image file to be processed Supported formats: JPG, PNG, BMP | |
ImageUrl | URL of the image to be processed Supported formats: JPG, PNG, BMP |
|
Unit | Unit of measurement for dimensions |
|
ImageWidth | Desired Width excluding frame, in AR | Any positive number. Decimals are supported, but fractions are not. |
ImageHeight | Desired Height excluding frame, in AR (Optional) | Any positive number. Decimals are supported, but fractions are not. |
MatWidth | Width of the mat (Optional) | Any positive number. Decimals are supported, but fractions are not. |
SupportType | Type of support |
|
FrameType | Type of frame |
|
FrameColor | Color of the frame (Optional) |
|
Available associations (frame type & color)
- Thin_Wood: available with
Black
,White
,Natural_Oak
- Large_Wood: available with
Gold
- Thin_Floater: available with
Black
,White
,Natural_Oak
- Floater: available with
Black
,White
,Natural_Oak
- Aluminum: available with
Black
,White
,Gold
,Silver
,Copper
REQUEST EXAMPLE
REQUEST | RESPONSE |
POST https://www.frameit.ar/api/frameit Content-Type: multipart/form-data Authorization: Basic api: your-API-key File: [image file] Unit: ImageWidth: MatWidth: SupportType: FrameType: FrameColor: | { "PublicLink": "https://public-link", "QrCodeImage": "https://qrcode-image" } |
Example of Javascript code
// Function to make the API request
async function uploadImage() {
// API endpoint
const url = 'https://www.frameit.ar/api/frameit';
// Prepare the form data
const formData = new FormData();
// Replace this with the URL of the image you want to use
const imageUrl = 'https://example.com/path/to/image.jpg';
formData.append('ImageUrl', imageUrl);
// Append other parameters to the form data
formData.append('Unit', 'in');
formData.append('ImageWidth', '24.5');
formData.append('MatWidth', '2');
formData.append('SupportType', 'Photo');
formData.append('FrameType', 'Thin_Wood');
formData.append('FrameColor', 'Natural_Oak');
// Set up the request headers
const headers = new Headers();
headers.append('Authorization', 'Basic ' + btoa('api:your-API-key'));
// Make the request
try {
const response = await fetch(url, {
method: 'POST',
headers: headers,
body: formData
});
if (!response.ok) {
throw new Error(HTTP error! status: ${response.status});
}
// Get the response JSON
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
// Call the function (e.g., on a button click)
uploadImage();
Example of Python code
import requests
from requests.auth import HTTPBasicAuth
import base64
# API endpoint
url = 'https://www.frameit.ar/api/frameit'
# Your API key
api_key = 'Your-API-Key'
def get_frameit_response():
# Image file path
file_path = 'images/sample1.jpg'
files = {'file': open(file_path, 'rb')}
# Data payload
data = {
'Unit': 'in',
'ImageWidth': 24.5,
'MatWidth': 2,
'SupportType': 'Photo',
'FrameType': 'Thin_Wood',
'FrameColor': 'Natural_Oak'
}
# Basic authentication
auth = HTTPBasicAuth('api', api_key)
# Make the POST request
response = requests.post(url, files=files, data=data, auth=auth)
# Print the response
print(response.json())
return response.json()
get_frameit_response()
HTTP Response Codes
- 200 OK: The request has succeeded and the response contains the requested data.
- 400 Bad Request: The request is malformed or contains incorrect parameters.
- 500 Internal Server Error: An error occurred on the server side.